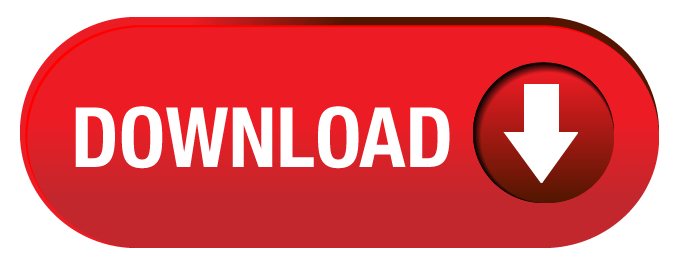
Overview
CMSIS Driver Implementations for the NXP LPC Microcontroller Series - ARM-software/NXPLPC 0, LPC4000 and LPC4300 under Apache 2.0 License. Signed-off-by: Joachim Krech. Generic COM-port devices and some modems belong to the abstract control model subclass. The protocol is V.25ter, which documents common AT commands. For compatibility with standard host drivers, a generic virtual COM-port device should specify the V.25ter protocol even if the device doesn’t use AT commands. Purpose: Port of Adafruit Device Driver for 3.2' 320x240 TFT LCD display to the NXP LPC1769 microprocessor using CooCox IDE. Original Author: Limor Fried (LadyAda) LPC1769 Port Author: James P. Target Board: NXP LPC1769 LPCXpresso evaluation board Software Development System: CooCox Eclipse-based free IDE. A more fully featured device, the PCAL9535A, is available with Agile I/O features. See the respective data sheet for more details. The PCA9535A open-drain interrupt (INT) output is activated when any input state differs from its corresponding Input Port register state and is used to indicate to the system controller that an input state has changed.
The MCUXpresso SDK provides Peripheral driver for the General Purpose I/O (GPIO) module of MCUXpresso SDK devices.
Initialization and deinitialization
The function GPIO_PinInit() initializes the GPIO with specified configuration.
Pin manipulation
The function GPIO_WritePinOutput() set output state of selected GPIO pin. The function GPIO_ReadPinInput() read input value of selected GPIO pin.
Port manipulation
Nxp Port Devices Drivers
The function GPIO_SetPinsOutput() sets the output level of selected GPIO pins to the logic 1. The function GPIO_ClearPinsOutput() sets the output level of selected GPIO pins to the logic 1. The function GPIO_TogglePinsOutput() reverse the output level of selected GPIO pins. The function GPIO_ReadPinsInput() read input value of selected port.
Port masking
The function GPIO_SetPortMask() set port mask, only pins masked by 0 will be enabled in following functions. The function GPIO_WriteMPort() sets the state of selected GPIO port, only pins masked by 0 will be affected. The function GPIO_ReadMPort() reads the state of selected GPIO port, only pins masked by 0 are enabled for read, pins masked by 1 are read as 0.
Example use of GPIO API. Refer to the driver examples codes located at <SDK_ROOT>/boards/<BOARD>/driver_examples/gpio
Data Structures |
struct | gpio_pin_config_t |
The GPIO pin configuration structure. More...
|
Enumerations |
enum | gpio_pin_direction_t { kGPIO_DigitalInput = 0U, kGPIO_DigitalOutput = 1U } |
LPC GPIO direction definition. More...
|
Functions |
static void | GPIO_PortSet (GPIO_Type *base, uint32_t port, uint32_t mask) |
Sets the output level of the multiple GPIO pins to the logic 1. More...
|
static void | GPIO_SetPinsOutput (GPIO_Type *base, uint32_t port, uint32_t mask) |
Sets the output level of the multiple GPIO pins to the logic 1. More...
|
static void | GPIO_PortClear (GPIO_Type *base, uint32_t port, uint32_t mask) |
Sets the output level of the multiple GPIO pins to the logic 0. More...
|
static void | GPIO_ClearPinsOutput (GPIO_Type *base, uint32_t port, uint32_t mask) |
Sets the output level of the multiple GPIO pins to the logic 0. More...
|
static void | GPIO_PortToggle (GPIO_Type *base, uint32_t port, uint32_t mask) |
Reverses current output logic of the multiple GPIO pins. More...
|
static void | GPIO_TogglePinsOutput (GPIO_Type *base, uint32_t port, uint32_t mask) |
Reverses current output logic of the multiple GPIO pins. More...
|
Driver version |
#define | FSL_GPIO_DRIVER_VERSION (MAKE_VERSION(2, 1, 1)) |
LPC GPIO driver version 2.1.1. More...
|
GPIO Configuration |
void | GPIO_PortInit (GPIO_Type *base, uint32_t port) |
Initializes the GPIO peripheral. More...
|
static void | GPIO_Init (GPIO_Type *base, uint32_t port) |
Initializes the GPIO peripheral. More...
|
void | GPIO_PinInit (GPIO_Type *base, uint32_t port, uint32_t pin, const gpio_pin_config_t *config) |
Initializes a GPIO pin used by the board. More...
|
GPIO Output Operations |
static void | GPIO_PinWrite (GPIO_Type *base, uint32_t port, uint32_t pin, uint8_t output) |
Sets the output level of the one GPIO pin to the logic 1 or 0. More...
|
static void | GPIO_WritePinOutput (GPIO_Type *base, uint32_t port, uint32_t pin, uint8_t output) |
Sets the output level of the one GPIO pin to the logic 1 or 0. More...
|
GPIO Input Operations |
static uint32_t | GPIO_PinRead (GPIO_Type *base, uint32_t port, uint32_t pin) |
Reads the current input value of the GPIO PIN. More...
|
static uint32_t | GPIO_ReadPinInput (GPIO_Type *base, uint32_t port, uint32_t pin) |
Reads the current input value of the GPIO PIN. More...
|
Data Structure Documentation

Every pin can only be configured as either output pin or input pin at a time. If configured as a input pin, then leave the outputConfig unused.
Data Fields |
gpio_pin_direction_t | pinDirection |
GPIO direction, input or output.
|
uint8_t | outputLogic |
Set default output logic, no use in input.
|
Macro Definition Documentation
#define FSL_GPIO_DRIVER_VERSION (MAKE_VERSION(2, 1, 1)) |
Enumeration Type Documentation
Enumerator |
---|
kGPIO_DigitalInput | Set current pin as digital input. |
kGPIO_DigitalOutput | Set current pin as digital output. |
Function Documentation

void GPIO_PortInit | ( | GPIO_Type * | base, |
uint32_t | port |
) |
This function ungates the GPIO clock.
Parametersbase | GPIO peripheral base pointer. |
port | GPIO port number. |
static void GPIO_Init | ( | GPIO_Type * | base, | uint32_t | port | ) |
| inlinestatic |
void GPIO_PinInit | ( | GPIO_Type * | base, |
uint32_t | port, |
uint32_t | pin, |
const gpio_pin_config_t * | config |
) |
To initialize the GPIO, define a pin configuration, either input or output, in the user file. Then, call the GPIO_PinInit() function.
This is an example to define an input pin or output pin configuration:
* gpio_pin_config_t config =
* kGPIO_DigitalInput,
* }
* gpio_pin_config_t config =
* kGPIO_DigitalOutput,
* }
Parametersbase | GPIO peripheral base pointer(Typically GPIO) |
port | GPIO port number |
pin | GPIO pin number |
config | GPIO pin configuration pointer |
static void GPIO_PinWrite | ( | GPIO_Type * | base, | uint32_t | port, | uint32_t | pin, | uint8_t | output | ) |
| inlinestatic |
Parametersbase | GPIO peripheral base pointer(Typically GPIO) |
port | GPIO port number |
pin | GPIO pin number |
output | GPIO pin output logic level.- 0: corresponding pin output low-logic level.
- 1: corresponding pin output high-logic level.
|
static void GPIO_WritePinOutput | ( | GPIO_Type * | base, | uint32_t | port, | uint32_t | pin, | uint8_t | output | ) |
| inlinestatic |
static uint32_t GPIO_PinRead | ( | GPIO_Type * | base, | uint32_t | port, | uint32_t | pin | ) |
| inlinestatic |
ParametersNXP Port Devices Driver
base | GPIO peripheral base pointer(Typically GPIO) |
port | GPIO port number |
pin | GPIO pin number |
Return values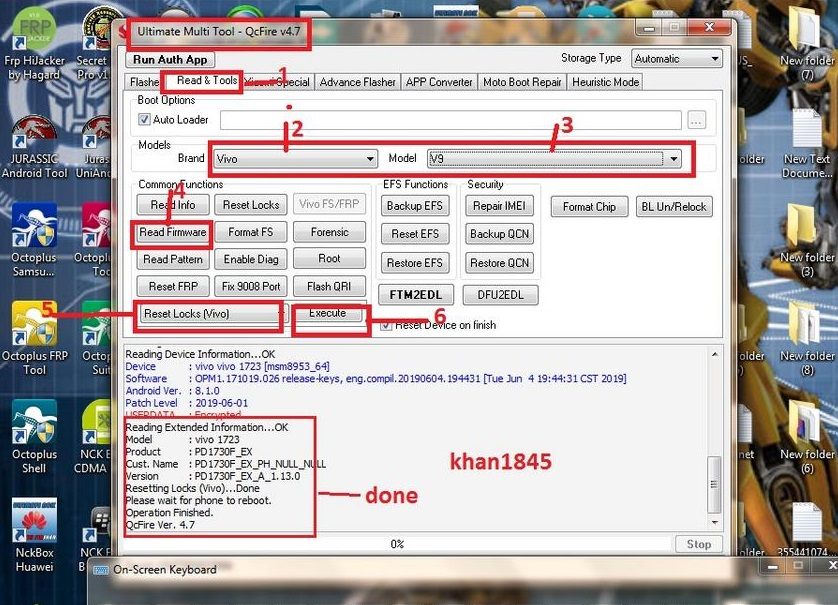
GPIO | port input value- 0: corresponding pin input low-logic level.
- 1: corresponding pin input high-logic level.
|
static uint32_t GPIO_ReadPinInput | ( | GPIO_Type * | base, | uint32_t | port, | uint32_t | pin | ) |
| inlinestatic |
static void GPIO_PortSet | ( | GPIO_Type * | base, | uint32_t | port, | uint32_t | mask | ) |
| inlinestatic |
Parametersbase | GPIO peripheral base pointer(Typically GPIO) |
port | GPIO port number |
mask | GPIO pin number macro |
static void GPIO_SetPinsOutput | ( | GPIO_Type * | base, | uint32_t | port, | uint32_t | mask | ) |
| inlinestatic |
static void GPIO_PortClear | ( | GPIO_Type * | base, | uint32_t | port, | uint32_t | mask | ) |
| inlinestatic |
Parametersbase | GPIO peripheral base pointer(Typically GPIO) |
port | GPIO port number |
mask | GPIO pin number macro |
static void GPIO_ClearPinsOutput | ( | GPIO_Type * | base, | uint32_t | port, | uint32_t | mask | ) |
| inlinestatic |
static void GPIO_PortToggle | ( | GPIO_Type * | base, | uint32_t | port, | uint32_t | mask | ) |
| inlinestatic |
Parametersbase | GPIO peripheral base pointer(Typically GPIO) |
port | GPIO port number |
mask | GPIO pin number macro |
static void GPIO_TogglePinsOutput | ( | GPIO_Type * | base, | uint32_t | port, | uint32_t | mask | ) |
| inlinestatic |